Dependencies
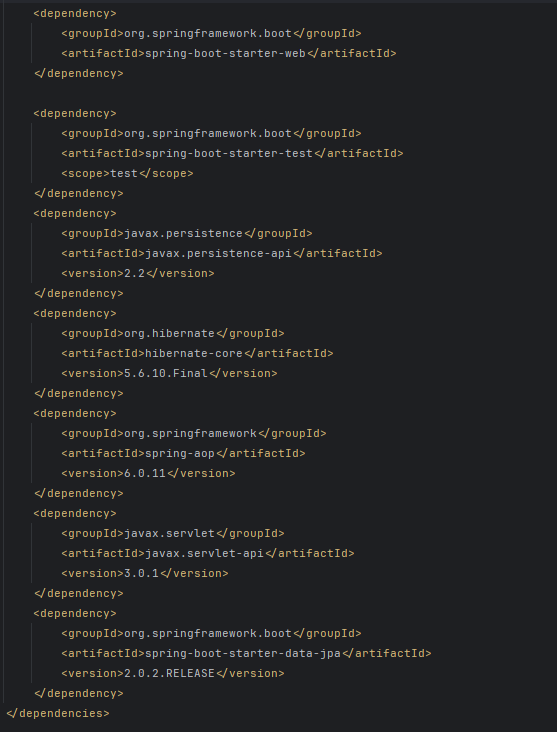
pom.xml
Dependencies, this project is a RESTful web service that: Exposes RESTful endpoints for various operations. Uses Spring Boot to streamline setup and configuration. Interacts with a relational database using JPA and Hibernate for ORM. Handles cross-cutting concerns with AOP. Is tested using Spring Boot's testing framework. Runs on an embedded server provided by Spring Boot.
Spring Boot Project Structure
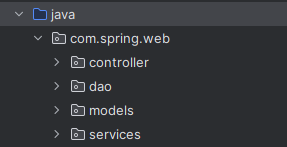
The Directories you listed often serve different purposes.
1.controller: This directory usually contains classes responsible for handling incoming HTTP requests, processing them, and returning an appropriate HTTP response. Controllers in a Spring Boot application are annotated with @Controller, @RestController, @RequestMapping, or similar annotations.
2. dao: This directory typically holds classes related to data access. DAO stands for Data Access Object, and it's a design pattern used to separate the business logic from the persistence layer (database operations). Classes in this directory often contain methods to interact with the database, perform CRUD (Create, Read, Update, Delete) operations, and execute queries.
3. models: This directory usually contains classes that represent the domain model of the application. These classes define the structure of the data that the application works with. They often map directly to database tables or other data sources. In a Spring Boot application, these classes are commonly annotated with JPA (Java Persistence API) annotations or other metadata to specify how they should be stored in the database.
4. services: This directory typically holds classes that contain the business logic of the application. These classes encapsulate the application's functionality and are responsible for coordinating interactions between controllers, DAOs, and models. Services in a Spring Boot application are often annotated with @Service to indicate that they should be automatically discovered and instantiated by the Spring framework's component scanning mechanism.
Packages and Classes
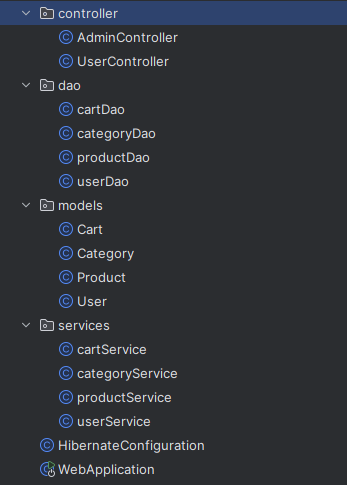
Each package and Classes serves a specific purpose
1. Controller: This package contains classes responsible for handling incoming HTTP requests and providing appropriate responses. In your case, you have AdminController and UserController, which likely handle requests related to administrative actions and user-related actions, respectively.
2. Dao: This package typically contains classes responsible for interacting with the database. The classes here are often referred to as Data Access Objects (DAOs). In your project, you have cartDao, categoryDao, productDao, and userDao, which likely contain methods for accessing and manipulating data related to carts, categories, products, and users in the database.
3. models: This package contains classes representing the domain model of your application. These classes typically map to database tables or other data structures in your application. In your project, you have Cart, Category, Product, and User classes, which likely represent entities in your application such as shopping carts, product categories, products, and users.
4. services: This package contains classes that encapsulate the application's business logic. These classes orchestrate interactions between controllers, DAOs, and other components. In your project, you have cartService, categoryService, productService, and userService classes, which likely contain methods implementing business logic related to carts, categories, products, and users.
5. HibernateConfiguration: This class likely contains configuration settings for Hibernate, an ORM (Object-Relational Mapping) framework commonly used with Spring projects. It typically includes settings related to database connection, session management, and entity mapping.
6. WebApplication: This class likely serves as the entry point of your Spring Boot application. It contains the main method and is responsible for bootstrapping the application context and starting the embedded web server
application.properties

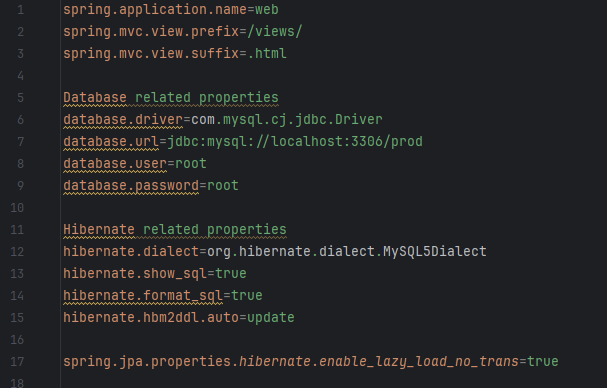
Breakdown of what each section of application.properties
1) Spring Application Properties: * spring.application.name: Sets the name of the Spring application context. It's often used for identifying your application in logs, metrics, etc. * spring.mvc.view.prefix: Defines the prefix for Spring MVC view names. In this case, it's set to /views/. * spring.mvc.view.suffix: Defines the suffix for Spring MVC view names. In this case, it's set to .html.
2) Database Related Properties: 2.1 database.driver: Specifies the JDBC driver class for connecting to the database. In this case, it's set to com.mysql.cj.jdbc.Driver. 2.2 database.url: Specifies the JDBC URL for connecting to the database. This URL defines the location of the database server and the name of the database. In this case, it's set to jdbc:mysql://localhost:3306/prod, indicating a MySQL database running on localhost with the database name prod. 2.3 database.user: Specifies the username for connecting to the database. 2.4 database.password: Specifies the password for connecting to the database.
3) Hibernate Related Properties: 3.1 hibernate.dialect: Specifies the SQL dialect to use. Hibernate uses this to generate appropriate SQL statements for the configured database. In this case, it's set to org.hibernate.dialect.MySQL5Dialect, indicating MySQL 5.x dialect. 3.2 hibernate.show_sql: Controls whether Hibernate should output the generated SQL to the console. In this case, it's set to true. 3.3 hibernate.format_sql: Controls whether Hibernate should format the generated SQL. In this case, it's set to true. 3.4 hibernate.hbm2: The property seems to be incomplete (hibernate.hbm2), but it's typically used for configuring Hibernate's HBM2DDL feature, which can automatically generate database schema from your Hibernate mappings.
Pages
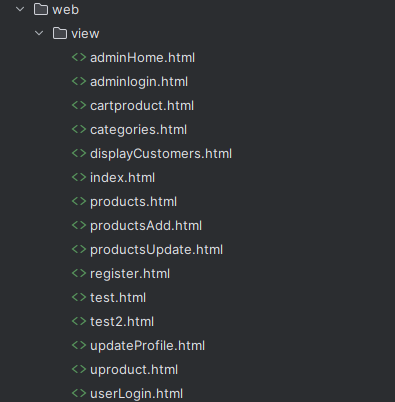
HTML files
HTML files create the web pages users see and interact with. They define how things look and where they go on the page, making it possible for users to use the application in their web browsers.
MySQL Workbench
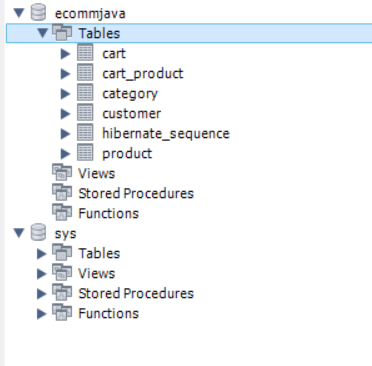
database
Tables:
Screenshots
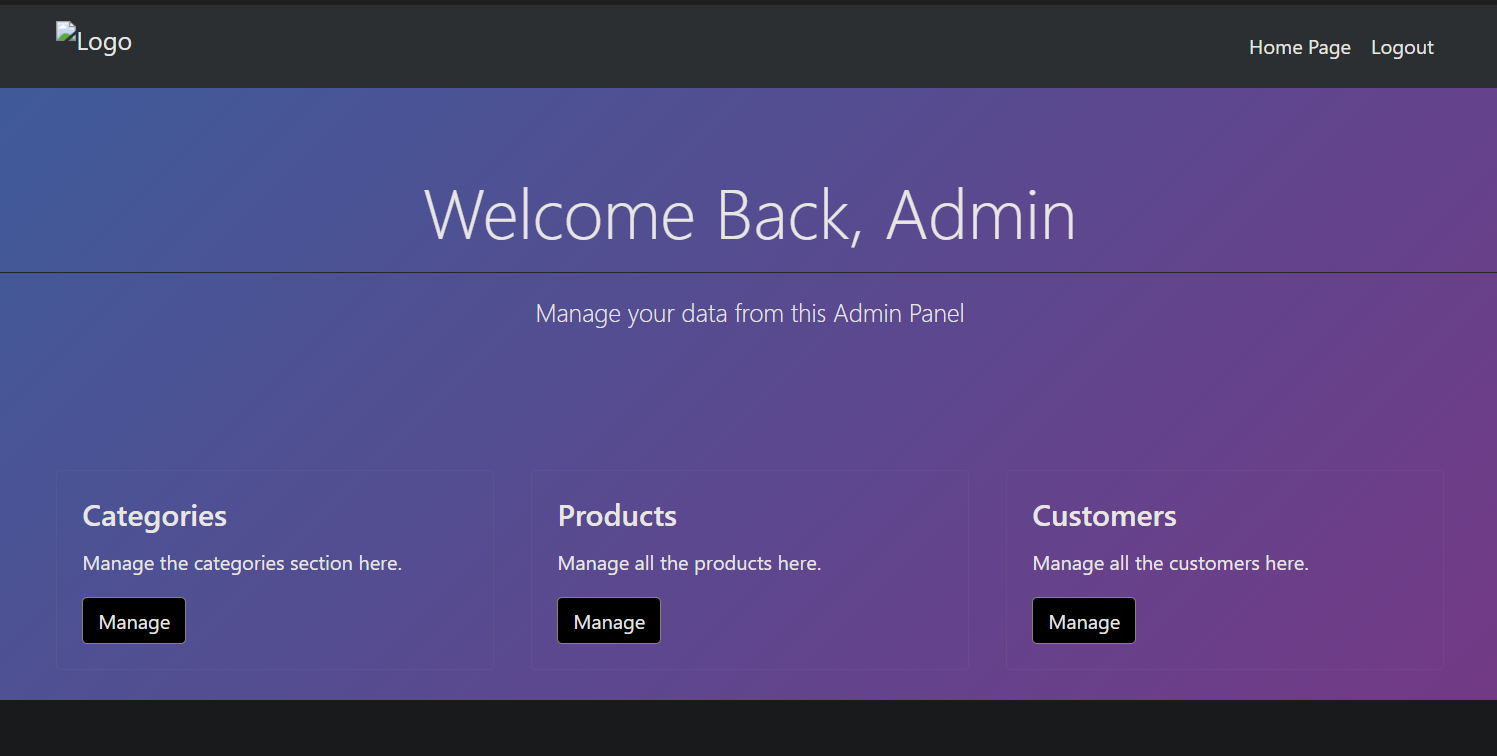
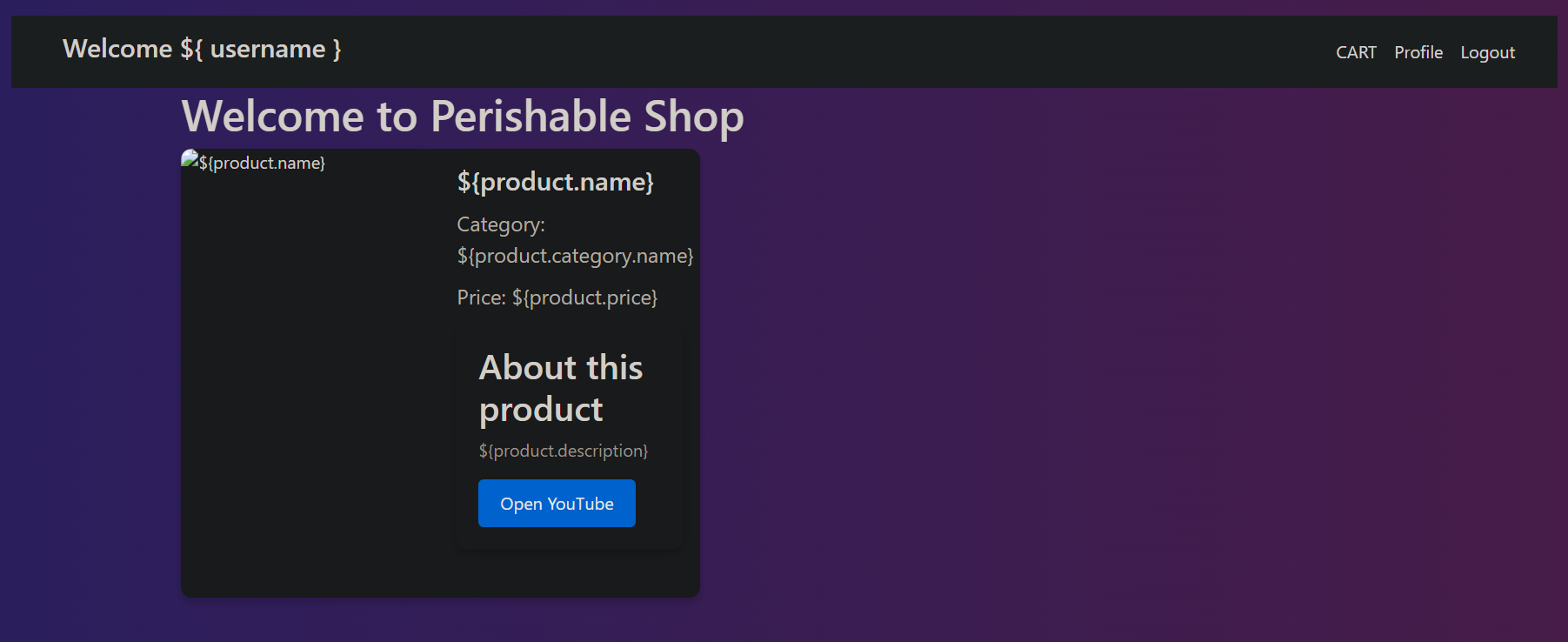
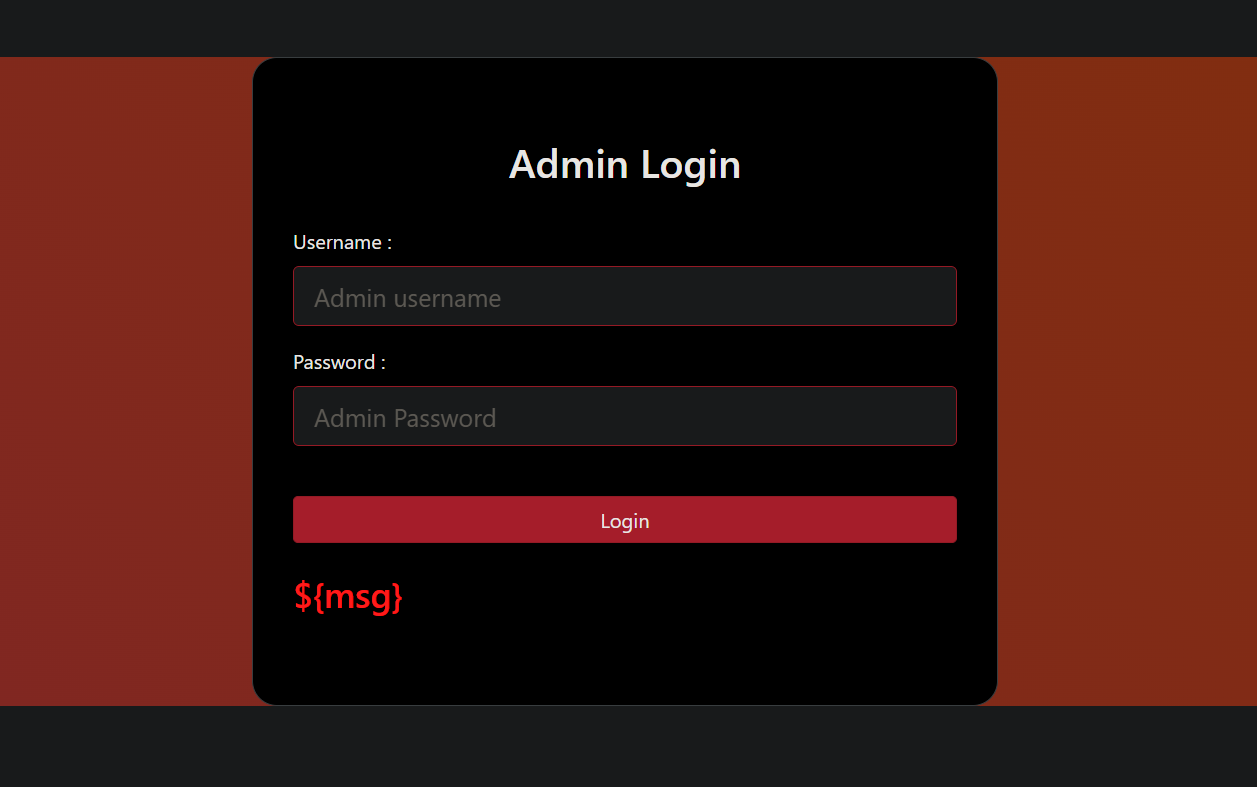
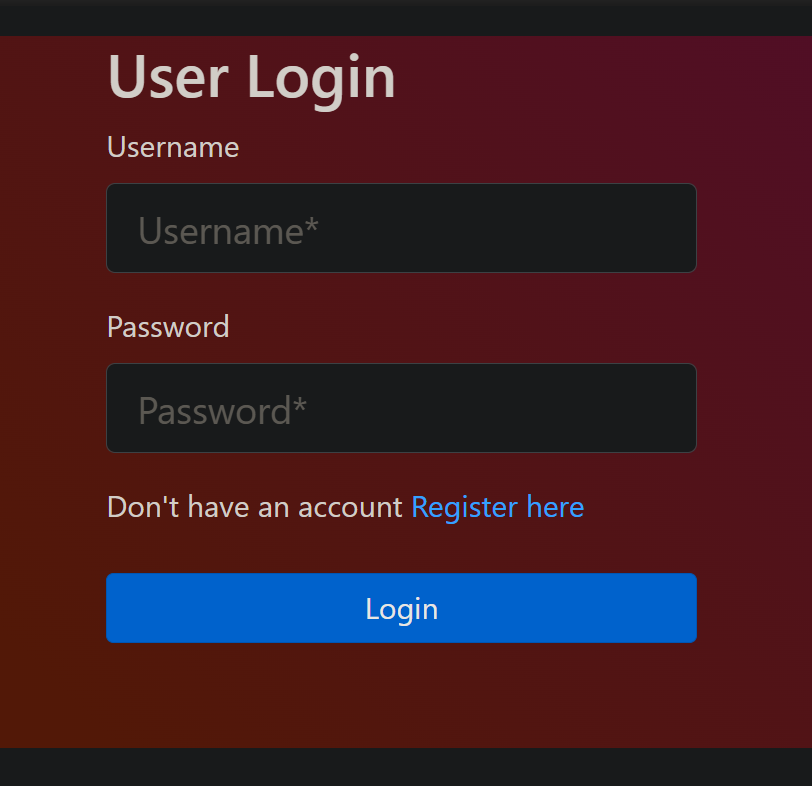
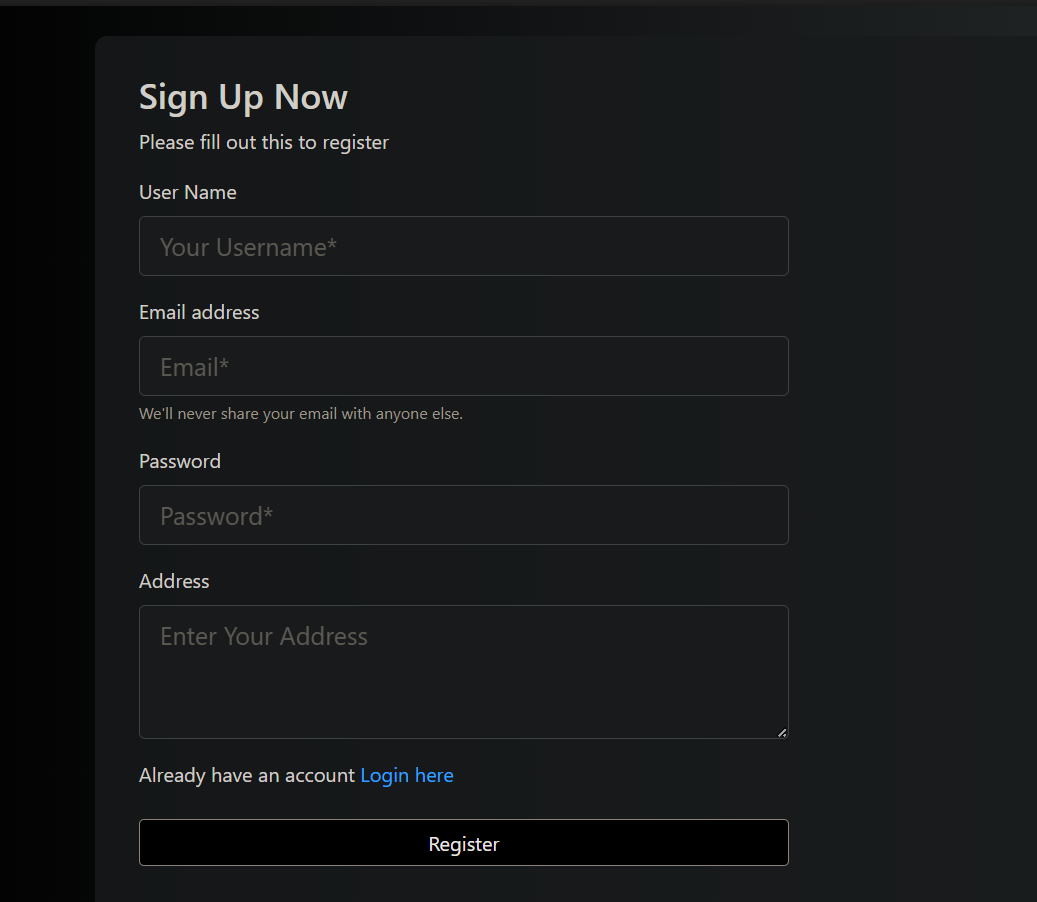
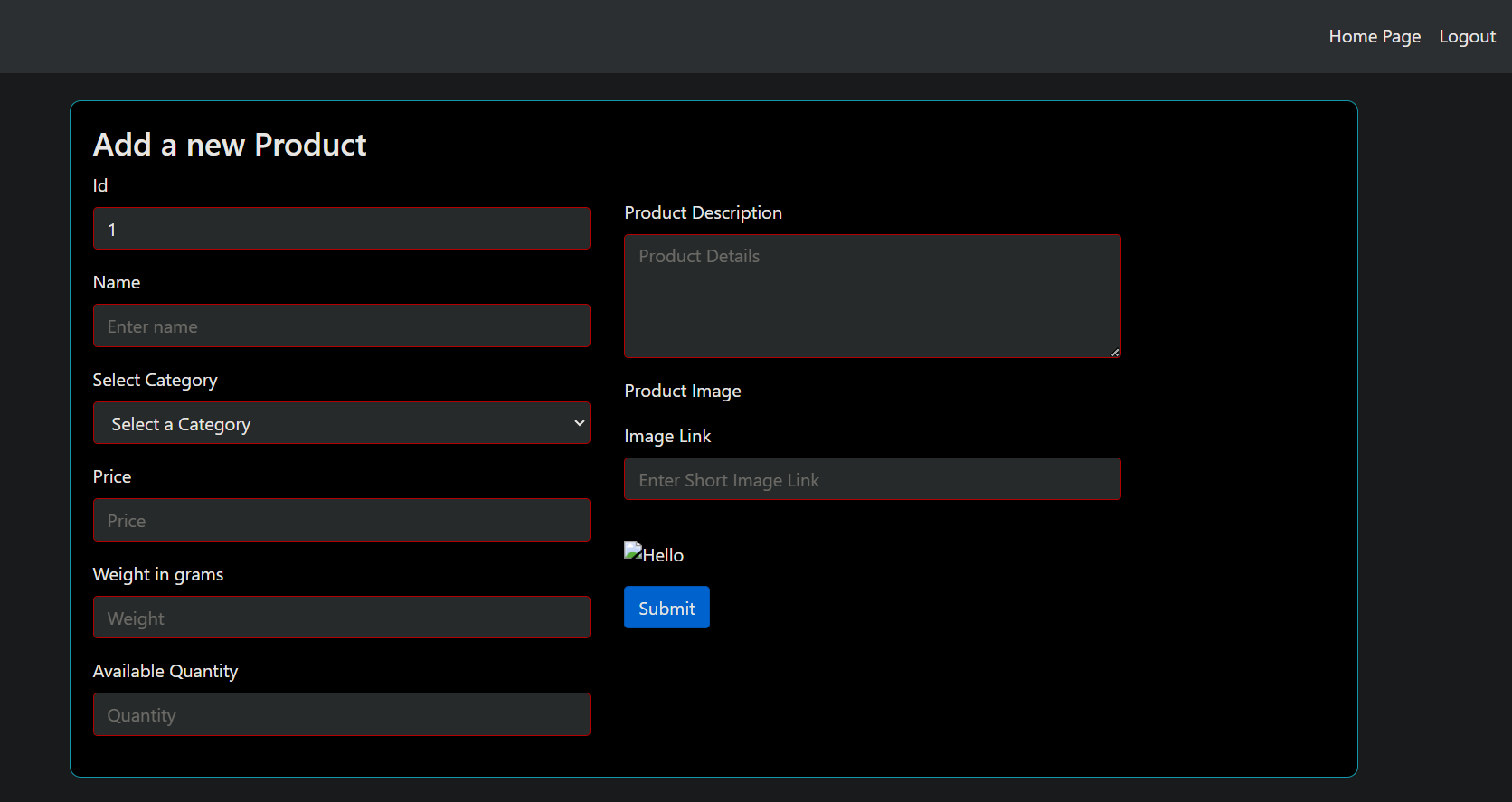